Gamifying your application is the process of adding game elements, such as user points and user achievements, inside your application. This will encourage your users to contribute and interact more in order to earn points and badges.
Luckily if you are using Laravel, there is a new package called laravel-gamify, where you can easily add gamification features to your application!
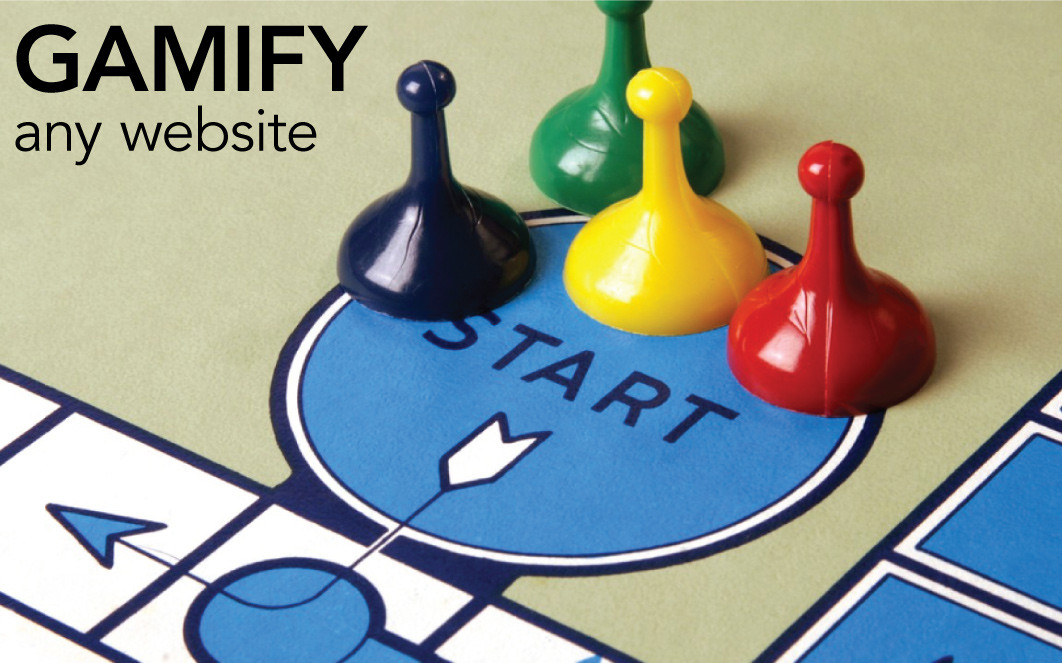
Ready to add some gamification to your application? Let's learn how easy it is to use this package. (you will need to use an existing laravel application or create a new one before proceeding)
1. Installing the package via Composer
First, we'll run the following composer command to install the package:
composer require qcod/laravel-gamify
2. Register the Service Provider (Skip if using Laravel 5.5 or greater)
You can register the Service Provider by adding it in config/app.php providers array:
'providers' => [
//...
QCod\Gamify\GamifyServiceProvider::class
]
In Laravel 5.5 and above the service provider is added automatically, so if you are using Laravel 5.5 or greater you can skip this step.
3. Publish and Run the Migrations
Next we'll want to publish the migrations:
php artisan vendor:publish --provider="QCod\Gamify\GamifyServiceProvider" --tag="migrations"
After our migrations have been published we will want to run those migrations:
php artisan migrate
and now our gamification tables will be added to our application.
4. Publish the Gamification config (optional)
This step is optional, but it will be helpful if you want to make changes to your gamification settings. To publish the config file, you can run the following command:
php artisan vendor:publish --provider="QCod\Gamify\GamifyServiceProvider" --tag="config"
That's it! We are now ready to use the Laravel Gamify package in our application.
5. Creating Points
To create a new point for our users to earn, we can use the following artisan command:
php artisan gamify:point PostCreated
This will create a new PointType class named PostCreated inside the app/Gamify/Points/ folder:
<?php
namespace App\Gamify\Points;
use QCod\Gamify\PointType;
class PostCreated extends PointType
{
/**
* Number of points
*
* @var int
*/
public $points = 20;
/**
* Point constructor
*
* @param $subject
*/
public function __construct($subject)
{
$this->subject = $subject;
}
/**
* User who will be receive points
*
* @return mixed
*/
public function payee()
{
return $this->getSubject()->user;
}
}
6. Assigning Points
In order to assign points we can use the new Gamify trait by adding it to any model in our application
use QCod\Gamify\Gamify;
use Illuminate\Notifications\Notifiable;
use Illuminate\Foundation\Auth\User as Authenticatable;
class User extends Authenticatable
{
use Notifiable, Gamify;
Now inside of a Controller where we want to award user points for creating a new article we can do the following:
$user = $request->user();
$post = $user->posts()->create($request->only(['title', 'body']));
// you can use helper function
givePoint(new PostCreated($post));
// or via HasReputation trait method
$user->givePoint(new PostCreated($post));
in some cases you may also want to undo a point, for example, the user deletes a post:
// via helper function
undoPoint(new PostCreated($post));
$post->delete();
// or via HasReputation trait method
$user->undoPoint(new PostCreated($post));
$post->delete();
You may also wish to get the total number of points per user:
// get integer point
$user->getPoints(); // 20
// formatted result
$user->getPoints(true); // if point is more than 1000 1K+
You can also assign Achievement Badges to users based on the amount of points they have. Be sure to learn more about this awesome package on their Github page at: https://github.com/qcod/laravel-gamify
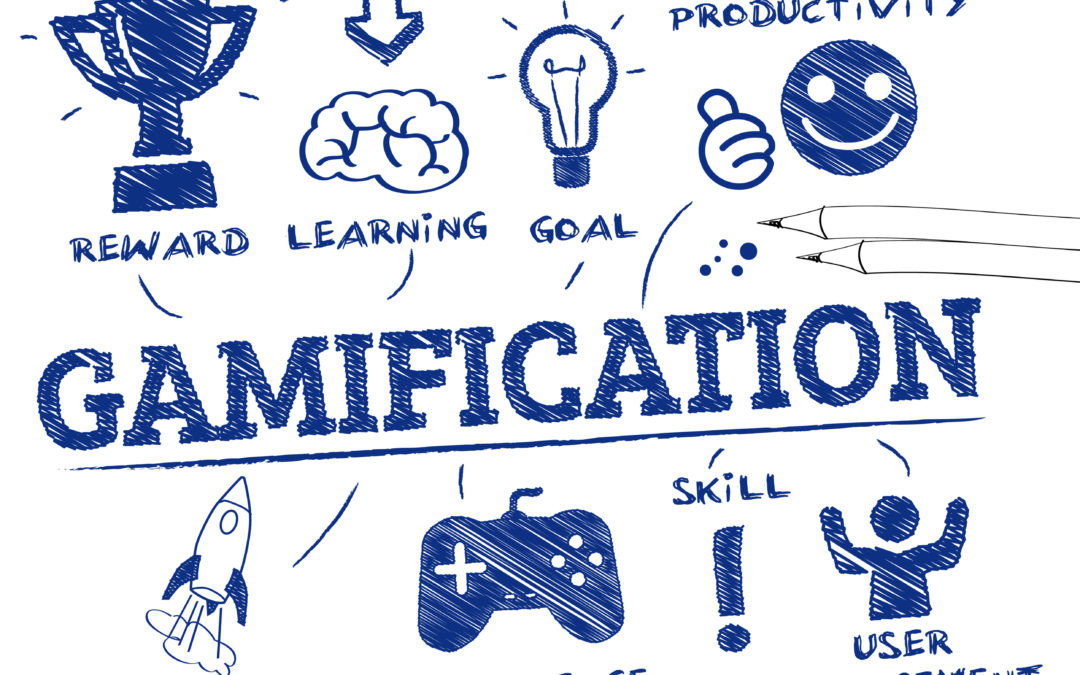
Be sure to download this package and include some awesome gamification features in your next Laravel application.